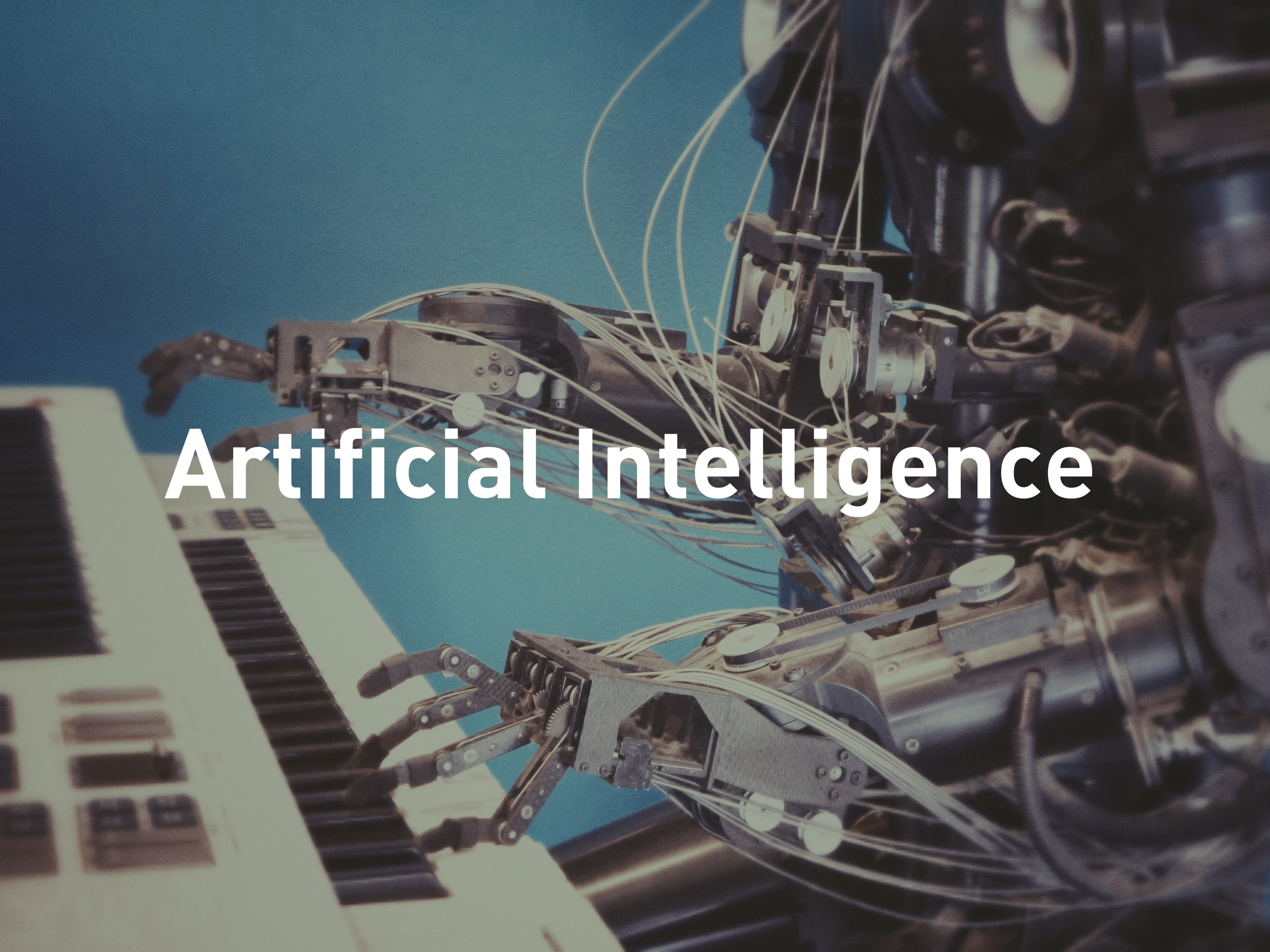
Description
In the course AI programming at DAE we learned a bunch of different techniques to include AI in our games.
We started off creating some very basic steering behaviours, which we then later built upon to create more complex behaviours, using blended & combined steering behaviours. Obviously, we needed to optimize this a little bit as well, that’s why we also saw how to use flocking & partitioning.
Eventually, we went and had a look at some different algorithms for pathfinding, the most important ones being the A* algorithm, together with the navigation meshes.
Eventually, we also saw a few different state machines, such as the finite-state machine and a behaviour tree.
At last, we had a small introduction to machine learning.
For the exam we then had to combine all of these together to create an AI for a zombie game, the goal of this bot was to survive as long as possible, whilst scoring the most points. (collecting items, killing zombies, etc.)
On top of this, we also had to choose 1 topic about AI programming. We then had to research this topic and describe it on the Github page.
You can find the classes’ source files on this GitHub page.
Engine: Custom C++ Framework (DAE)
Topics
SteeringBehaviours
We started off creating some very basic steering behaviours.
Here’s a list of all base behaviours:
- Seek
- Flee
- Wander
- Pursuit
- Evade
Flocking/Partitioning
After creating a bit more complex steering behaviours such as:
- Cohesion - Seek the average position of neighbours
- Separation - Flee from the average position of neighbours
- VelocityMatch - Match the average velocity of neighbour agents
We were able to create some really cool looking flocking effect with the use of blended steering behaviours.
However, to get these cool outcomes we needed to have a lot of agents. To be able to run this project smoothly we also implemented Spatial Partitioning.
Pathfinding
As for pathfinding, we started off by creating the very popular A* algorithm. Before we implemented A* itself we first saw how to use the Dijkstra algorithm.
In this example, the water is not traversable at all, while the mud is, but it comes at a higher cost.
Navigation Mesh
The next part of the course was a navigation mesh.
In short, this is a mesh where the actors can traverse on.
Using A* we then made it possible to calculate the shortest path to the target on this navmesh.
State Machines / BehaviourTrees
We also had to use Finite State Machines & Behaviour Trees.
This example to the right is actually my exam project, in this project I mainly used behaviour trees. Obviously, based on what the agent can see, his behaviour will change. For example, if he sees a wall, he’ll try to find the entrance of that building. But if he sees an enemy and has no gun, he’ll try and evade that enemy.
Research Topic: Context Steering
For my research topic, I chose to have a deeper look into context steering. In my example, I used it mainly for obstacle avoidance.
If you want to have a more depth explanation, please have a look at it’s Github page.